Introduction :
Seeder is a unique feature or method in Laravel that is used to generate or store dummy data in the database. Seeder is make with one command. Any number of static data can be created in seeder and multiple seeders can be created. Many times the database is refreshed or rolled back, even after that the test data can be generated anytime by seeding the seeder. Even after the data is deleted, the data remains in the seeder, only the data is permanently deleted from the table.
Table of content :
Make seeder with command
Create data into the seeder
Run single seeder seed command
Add class in database\seeders\DatabaseSeeder.php
Run multiple seeder with seed command
1. Make seeder : see command and code below :
php artisan make:seeder UserSeeder
database\seeders\UserSeeder.php
<?php namespace Database\Seeders; use Illuminate\Database\Seeder; class UserSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { // } }
2. Create data into the seeder :
There is a run function inside the seeder, inside which we have to create the data. You can create data with any number of rows in insert function.
Look code below :
<?php namespace Database\Seeders; use Illuminate\Database\Seeder; use Illuminate\Support\Facades\DB; use Illuminate\Support\Facades\Hash; class UserSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { DB::table('users')->truncate(); DB::table('users')->insert([ ['name' => 'user1', 'email' => 'user1yopmail.com', 'password' => Hash::make('12345678')], ['name' => 'user2', 'email' => 'user2yopmail.com', 'password' => Hash::make('87654321')] ]); } }
3. Run single seeder with seed command
After data is added to the function, the seeder is seeded with commands so that the data is inserted into the tables of the database.
For example see the command and output image below:
php artisan db:seed UserSeeder
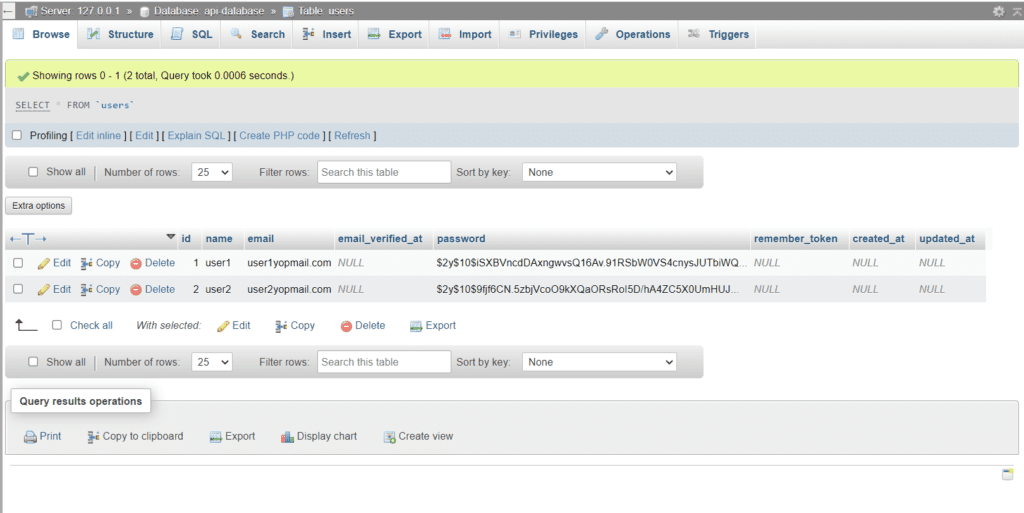
4. Add all seeder class in database\seeders\DatabaseSeeder.php
A specific seeder is also seeded, but if there are multiple seeders, to seed them together in the database, all the seeders are added to this file (Database\seeders\DatabaseSeeder.php) with the seeder class. A specific seeder is also seeded, but if there are multiple seeders, all seeders are associated with a seeder class in this file Database\seeders\DatabaseSeeder.php to seed them together in the database. After this, the seeder command is run so that all the seeder’s data is stored in the table with the running of one command.
For example look code below :
database\seeders\DatabaseSeeder.php
<?php namespace Database\Seeders; use Illuminate\Database\Seeder; class DatabaseSeeder extends Seeder { /** * Seed the application's database. * * @return void */ public function run() { // \App\Models\User::factory(10)->create(); $this->call(UserSeeder::class); $this->call(StudentSeeder::class); } }
5. Run multiple seeder with seed command
php artisan db:seed
Insert data with forcing seeders :
Seeders can also be seeded forced, for this the command is used. Forced means to insert data anytime without any interruption. See the below command :
php artisan db:seed –force